Thursday, June 29, 2006
jQuery Visual Documentation
Here is a link for the visual reference to jQuery, the super-lightweight library designed to make the most common JavaScript tasks easy and fun.
Tuesday, June 27, 2006
Lotus Notes categorized view Accordian using jQuery
This is another early SnTT. This is another follow up previous writeup on categorized views. Lotus Notes categorized view on web will require refresh for every expand or collapse of the view. To counter this, retrieve all the data and build our own view. In this example, I'm using jQuery instead.
I've create a simple example of building a Accordian style categorized view using jQuery. It retrieve the view xml - ReadViewEntries - and build the categorized view. It uses jQuery basic effects to slide up and down the content when expanding or collapsing the view.
1) Firstly include the jquery.js in the header. Download this viewfunction.js and add it into the header.
"<script type='text/javascript' src='/"+ @WebDbName +"/jquery.js'></script> <script type='text/javascript' src='/"+ @WebDbName +"/viewfunction.js'></script>"
2) Create a division name "viewdisplay". The generated view will be populated in this division.
<div id="viewdisplay">
3) In the JS Header add the code below. The jQuery $(document).ready(function(){}); event will be called the instant the DOM is ready to be manipulated. Pass in the view URL (?ReadViewEntry).
$(document).ready(function(){
getView("servername/dbpath/viewname?ReadViewEntries&ExpandView&Count=1000")
});
4) the getView function will built the view and display it in the "viewdisplay" division. For each category, it will append in the class "catcol<num>" (num starting form 0). Then for each column, it will append the class "col<num>" (num starting from 0). So you can format the look and feel for the view.
Example css for the view
.catcol0{clear:both;margin-left:20px;}
.catcol1{clear:both;margin-left:40px;}
.col0{clear:both;float:left;margin-left:60px;width:200px;}
Please take note that this is just a simple working example. It can generate single and multiple category. Make sure the view are expanded when using this (defeats the purpose if keep it collapse as the script will have to go to backend to retrieve the content). At the moment, the function still does not take into consideration of building views starting somewhere in the middle of the view. The slideUp and slideDown effects works nicely on Firefox. On IE, there will be some slight flicker. To overcome this, the page have to be renders in XHTML instead.
Download the js and tried it out. Appreciate any feedback on how and what to improve on that.
Updated (28/June/2006) - The viewfunction.js posted previously was not the working version. I've updated the js file.
Show-n-Tell Thursday
I've create a simple example of building a Accordian style categorized view using jQuery. It retrieve the view xml - ReadViewEntries - and build the categorized view. It uses jQuery basic effects to slide up and down the content when expanding or collapsing the view.
1) Firstly include the jquery.js in the header. Download this viewfunction.js and add it into the header.
"<script type='text/javascript' src='/"+ @WebDbName +"/jquery.js'></script> <script type='text/javascript' src='/"+ @WebDbName +"/viewfunction.js'></script>"
2) Create a division name "viewdisplay". The generated view will be populated in this division.
<div id="viewdisplay">
3) In the JS Header add the code below. The jQuery $(document).ready(function(){}); event will be called the instant the DOM is ready to be manipulated. Pass in the view URL (?ReadViewEntry).
$(document).ready(function(){
getView("servername/dbpath/viewname?ReadViewEntries&ExpandView&Count=1000")
});
4) the getView function will built the view and display it in the "viewdisplay" division. For each category, it will append in the class "catcol<num>" (num starting form 0). Then for each column, it will append the class "col<num>" (num starting from 0). So you can format the look and feel for the view.
Example css for the view
.catcol0{clear:both;margin-left:20px;}
.catcol1{clear:both;margin-left:40px;}
.col0{clear:both;float:left;margin-left:60px;width:200px;}
Please take note that this is just a simple working example. It can generate single and multiple category. Make sure the view are expanded when using this (defeats the purpose if keep it collapse as the script will have to go to backend to retrieve the content). At the moment, the function still does not take into consideration of building views starting somewhere in the middle of the view. The slideUp and slideDown effects works nicely on Firefox. On IE, there will be some slight flicker. To overcome this, the page have to be renders in XHTML instead.
Download the js and tried it out. Appreciate any feedback on how and what to improve on that.
Updated (28/June/2006) - The viewfunction.js posted previously was not the working version. I've updated the js file.
Show-n-Tell Thursday
Monday, June 26, 2006
jQuery
Recently I've been testing out jQuery. Why do I use jQuery instead of prototype / scriptaculous? The main reason is because of the size. The full version of the framework is just 16K.
From jQuery website
jQuery is designed to change the way that you write Javascript. jQuery is a Javascript library that takes this motto to heart: Writing Javascript code should be fun. jQuery acheives this goal by taking common, repetitive, tasks, stripping out all the unnecessary markup, and leaving them short, smart and understandable.
jQuery itself have most of the features that I need. For example DOM traversing and modification, event handling, iteration, style, effects and of course AJAX. All this is pack in a single js file of 16K.
I'll put up some of the features and function of using jQuery with Lotus Notes in the future. So lookup for the writeup.
From jQuery website
jQuery is designed to change the way that you write Javascript. jQuery is a Javascript library that takes this motto to heart: Writing Javascript code should be fun. jQuery acheives this goal by taking common, repetitive, tasks, stripping out all the unnecessary markup, and leaving them short, smart and understandable.
jQuery itself have most of the features that I need. For example DOM traversing and modification, event handling, iteration, style, effects and of course AJAX. All this is pack in a single js file of 16K.
I'll put up some of the features and function of using jQuery with Lotus Notes in the future. So lookup for the writeup.
Monday, June 19, 2006
Quick Tip - Web font family and font size in Lotus Notes
For web development in Lotus Notes, it is a good practise to use CSS to style page / form. This is true especially for the font-family and font-size.
Example, if you create a simple text page (example below). Change the font family to Arial and the font size to 9
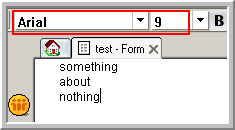
The sample html generated:
<font size="2" face="Arial">something</font><br><font size="2" face="Arial">about</font><br>
<font size="2" face="Arial">nothing</font>
If you have a big page / form, imagine the much overhead is generated. To overcome this, use font "Default Sans Serif" with size "10". Insert a css link in the HTML Head
"<link rel=\"stylesheet\" href=\"/" + @WebDbName + "/style.css\" type=\"text/css\" />"
Create a page name style.css . This link to previous writeup on extra css property settings. Insert the code below.
body{font-family:Arial;font-size:2}
The code will reduce to
something<br>
about<br>
nothing
This would help reduce to size of the page. If you have performance issue for the web pages, this is one of the way to help improve the performance (may or may not be major improvement depending on your page). Try it out :)
Show-n-Tell Thursday
Example, if you create a simple text page (example below). Change the font family to Arial and the font size to 9
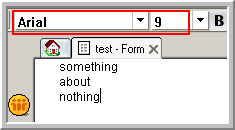
The sample html generated:
<font size="2" face="Arial">something</font><br><font size="2" face="Arial">about</font><br>
<font size="2" face="Arial">nothing</font>
If you have a big page / form, imagine the much overhead is generated. To overcome this, use font "Default Sans Serif" with size "10". Insert a css link in the HTML Head
"<link rel=\"stylesheet\" href=\"/" + @WebDbName + "/style.css\" type=\"text/css\" />"
Create a page name style.css . This link to previous writeup on extra css property settings. Insert the code below.
body{font-family:Arial;font-size:2}
The code will reduce to
something<br>
about<br>
nothing
This would help reduce to size of the page. If you have performance issue for the web pages, this is one of the way to help improve the performance (may or may not be major improvement depending on your page). Try it out :)
Show-n-Tell Thursday
Wednesday, June 14, 2006
Changing image on mouseover & mouseout
Usually we will create 2 seperate images for mouse over effects. In Lotus Notes you can do it by using 1 image only. Create one image containing the orginal image and mouse over image side by side. Add a line seperator in the middle. Sample image below.
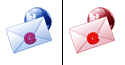
Create a new image resource. In the property "Basic" tab, change the value of "Image cross" to 2.
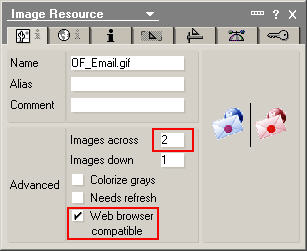
In your form, you can insert the image resource. By default, Lotus Notes will show the first image (Left). When previewing the form, the image will change to the second image(right) when mouseover the image and back to the first image when mouseout.
This works on web also. In the image property, check on "Web browser compatible". Domino will create the function below.
<script language="JavaScript" type="text/javascript">
<!--
function dominoWellLoad(elem, baseUrl, width) {
elem.well = new Array();
for (var i=0; i < width; i++) {
elem.well[i] = new Image();
elem.well[i].src = baseUrl+"&ImgIndex="+i;
}
}
// -->
</script>
<img src="dbpath.nsf/Email.gif?OpenImageResource&ImgIndex=0" width="62" height="65" onmouseover="if (this.well) this.src = this.well[1].src;" onmouseout="if (this.well) this.src = this.well[0].src;" onload="if(null==this.well) dominoWellLoad(this, 'dbpath.nsf/Email.gif?OpenImageResource&ImgIndex=0', 2);" alt="">
You can also use the image individually by from the source
<dbpath>/<imagename>?OpenImageResource&ImgIndex=0
or
<dbpath>/<imagename>?OpenImageResource&ImgIndex=1
By default, the cursor remains the same so you may want to add to the style or a class in stylesheet to change it to a pointer.
{cursor:pointer}
You can try out using more image
2 (Second position) - for mouseover
3 (Third positio) - for selected image
4 (Fourth position) - for mouse-down image
Show-n-Tell Thursday
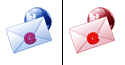
Create a new image resource. In the property "Basic" tab, change the value of "Image cross" to 2.
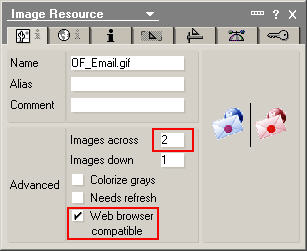
In your form, you can insert the image resource. By default, Lotus Notes will show the first image (Left). When previewing the form, the image will change to the second image(right) when mouseover the image and back to the first image when mouseout.
This works on web also. In the image property, check on "Web browser compatible". Domino will create the function below.
<script language="JavaScript" type="text/javascript">
<!--
function dominoWellLoad(elem, baseUrl, width) {
elem.well = new Array();
for (var i=0; i < width; i++) {
elem.well[i] = new Image();
elem.well[i].src = baseUrl+"&ImgIndex="+i;
}
}
// -->
</script>
<img src="dbpath.nsf/Email.gif?OpenImageResource&ImgIndex=0" width="62" height="65" onmouseover="if (this.well) this.src = this.well[1].src;" onmouseout="if (this.well) this.src = this.well[0].src;" onload="if(null==this.well) dominoWellLoad(this, 'dbpath.nsf/Email.gif?OpenImageResource&ImgIndex=0', 2);" alt="">
You can also use the image individually by from the source
<dbpath>/<imagename>?OpenImageResource&ImgIndex=0
or
<dbpath>/<imagename>?OpenImageResource&ImgIndex=1
By default, the cursor remains the same so you may want to add to the style or a class in stylesheet to change it to a pointer.
{cursor:pointer}
You can try out using more image
2 (Second position) - for mouseover
3 (Third positio) - for selected image
4 (Fourth position) - for mouse-down image
Show-n-Tell Thursday
JSON for Dummies part 2
Below are clipping from My Portal Project, JSON for Dummies part 2:
Change the JSON response so that we have a method called “actionToTake”, and call it directly:
{"ajaxReturn":
{"actionToTake" :
function(){
alert('The description and category fields are required.');
}
}
}
In the above sample we created the “actionToTake” object as a function, with no parameters. Now we can use the following code to process our action:
function processJsonResponse(originalRequest){
var domText = originalRequest.responseText;
var jsonResult = eval("(" + domText + ")");
jsonResult.ajaxReturn.actionToTake();
}
This is another example of JSON strength.
Change the JSON response so that we have a method called “actionToTake”, and call it directly:
{"ajaxReturn":
{"actionToTake" :
function(){
alert('The description and category fields are required.');
}
}
}
In the above sample we created the “actionToTake” object as a function, with no parameters. Now we can use the following code to process our action:
function processJsonResponse(originalRequest){
var domText = originalRequest.responseText;
var jsonResult = eval("(" + domText + ")");
jsonResult.ajaxReturn.actionToTake();
}
This is another example of JSON strength.
Tuesday, June 13, 2006
JSON for Dummies
So what is JSON all about? How do you actually pronounce it? Is it 'J' 'S' 'O' 'N'? I personally call it J'a'SON.
Jeff Crossett at My Portal Project wrote an article on JSON for Dummies (like me) Part 1. Check it out here.
Jeff Crossett at My Portal Project wrote an article on JSON for Dummies (like me) Part 1. Check it out here.
Wednesday, June 07, 2006
InViewEdit on web
In my recent application, there are a few views that only have only a few columns (2-4 columns). In Lotus Notes client, you will have the InViewEdit feature. Since the most of the data are being be edited is in the view directly, I mimic the InViewEdit function for web views.
I've created a sample database to showcase this. I use "?ReadViewEntries" and prototype / AJAX to retrieve the XML viewentries. Another way to do it is to directly generate the html in the view itself. To keep things simple, in the view, the column name is the same and the fieldname. The form "Main" will retrieve the view and display it.
First create the layout for the view. From the XML, I traverse each viewentry and use "div" to generate each role each row. Each column data have their own id.
<div>
<span class="col0" id="e_0_0">William Be</span>
<span class="col1" id="e_0_1">012-3456789</span>
<span id="button0"><img class="editbutton" onclick="editEntry('0', '8F6')" src="pencil.jpg"></span>
</div>
Below is the sample result:
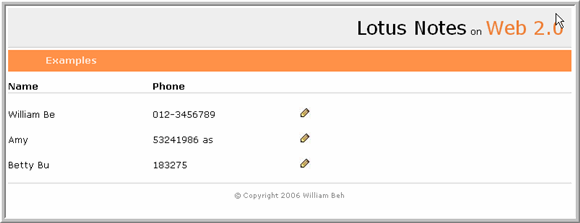
I've added button/image which will call the function to edit the row. It will pass the row number that is going to be edited and the notesid of the document. Textbox will be generated on the row. Then the button/image will change to confirm or cancel update.
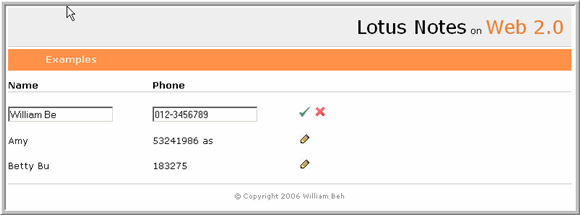
Cancel will restore the original value (remove the textbox). Update will do an AJAX POST to an agent. The agent will update the appropriate document. Then it will return success or error message. If there's an error, the original value will be restored. If the agent return success status, the update value will be displayed (removing the textbox).
In the agent, instead of using XML for the return message, I use JSON. Sample JSON message
{status:"error",
msg:"Doc not found"}
In the javascript, use eval to set the JSON object into a variable. Check if there is an error, alert the user and exit the function. If not error, it will continue processing.
var data = eval('(' + req.responseText + ')');
if(data.status == "error"){
alert(data.msg);
resetEntry(eleNum, noteid);
return;
}
I prefer to use JSON because I find it easier to traverse the data compare to XML where more coding is needed.
The detail codes is in the sample database. You can download it here.
This is just a simple example. Using in view editing will definitely reduce some steps need to update data in documents. But there are things that need to be taken into consideration.
First is in view editing by multiple users. What will happen when one editor update the data, But another editor is at the same page which is still show the old data? What will happen is the second editor to edit the same document. I am working on implementing some checking for this. I will try to publish that when it's done. Drop me a line if you have any suggestions on this also.
In this example, it only uses text box. I will improve it by adding selection, date, name lookup, etc.
Definitely you will have to include some form of validation before posting the update. You won't want user to accidentally clear the field and save the update.
Anyway, download the sample and test it out. Drop me a line if you have any suggestion on improving it.
Show-n-Tell Thursday
I've created a sample database to showcase this. I use "?ReadViewEntries" and prototype / AJAX to retrieve the XML viewentries. Another way to do it is to directly generate the html in the view itself. To keep things simple, in the view, the column name is the same and the fieldname. The form "Main" will retrieve the view and display it.
First create the layout for the view. From the XML, I traverse each viewentry and use "div" to generate each role each row. Each column data have their own id.
<div>
<span class="col0" id="e_0_0">William Be</span>
<span class="col1" id="e_0_1">012-3456789</span>
<span id="button0"><img class="editbutton" onclick="editEntry('0', '8F6')" src="pencil.jpg"></span>
</div>
Below is the sample result:
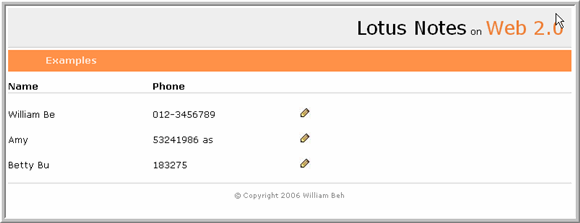
I've added button/image which will call the function to edit the row. It will pass the row number that is going to be edited and the notesid of the document. Textbox will be generated on the row. Then the button/image will change to confirm or cancel update.
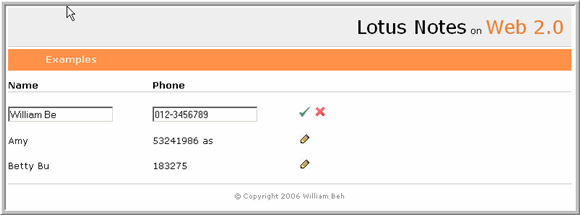
Cancel will restore the original value (remove the textbox). Update will do an AJAX POST to an agent. The agent will update the appropriate document. Then it will return success or error message. If there's an error, the original value will be restored. If the agent return success status, the update value will be displayed (removing the textbox).
In the agent, instead of using XML for the return message, I use JSON. Sample JSON message
{status:"error",
msg:"Doc not found"}
In the javascript, use eval to set the JSON object into a variable. Check if there is an error, alert the user and exit the function. If not error, it will continue processing.
var data = eval('(' + req.responseText + ')');
if(data.status == "error"){
alert(data.msg);
resetEntry(eleNum, noteid);
return;
}
I prefer to use JSON because I find it easier to traverse the data compare to XML where more coding is needed.
The detail codes is in the sample database. You can download it here.
This is just a simple example. Using in view editing will definitely reduce some steps need to update data in documents. But there are things that need to be taken into consideration.
First is in view editing by multiple users. What will happen when one editor update the data, But another editor is at the same page which is still show the old data? What will happen is the second editor to edit the same document. I am working on implementing some checking for this. I will try to publish that when it's done. Drop me a line if you have any suggestions on this also.
In this example, it only uses text box. I will improve it by adding selection, date, name lookup, etc.
Definitely you will have to include some form of validation before posting the update. You won't want user to accidentally clear the field and save the update.
Anyway, download the sample and test it out. Drop me a line if you have any suggestion on improving it.
Show-n-Tell Thursday
Tuesday, June 06, 2006
Form Validator
Friday, June 02, 2006
Serving JavaScript Fast
Here's an interesting article by Cal Henderson on Serving JavaScript Fast
Quote from the article
The next generation of web apps make heavy use of JavaScript and CSS. We’ll show you how to make those apps responsive and quick.
Quote from the article
The next generation of web apps make heavy use of JavaScript and CSS. We’ll show you how to make those apps responsive and quick.
Thursday, June 01, 2006
@Formula using Ajax
There have been quite a lot of articles written on how to call @Formula using Ajax. Below are two sample from searchdomino.
1. DBColumn and DBLookup for all browsers using AJAX
2. Using AJAX as a replacement for @DBLookups
I'm developing an application which needs uses quite a lot of formula (especially @DBLookup & @UserRoles of different selected person) at the client side. To port it to web, I need to use those too.
Instead of creating new agents for each @Formula, I created a formula setup Form where I can enter different formula. Each document will have a unique title (single word). I use tags (<keyn>) that will be replace by parameter pass in through url.

Using a view, I use a different form to open the document. This is set in the "Form Formula". The new form will compute the formula and return the result. In the view, set the title as the first column and sort it either ascending or descending. This way you can get the document using the title instead of document unique id
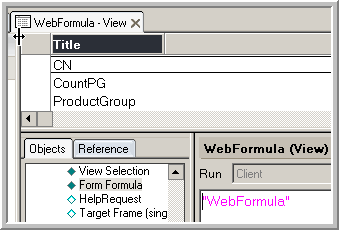
Below is a sample where different product group is selected, the products will be retrieved (DBLookup) and populated into the Product selection list. Check out previous post on dynamic selection list.

I use AJAX to call the formula document
<dbpath>/vWebFormula/<formula_document_title>?OpenDocument¶m=key1~value1
The param is optional. That will return the result in text format. You can then process it as needed. If you need more formula just create a new setup formula document and you are ready to use it. You can download the sample db here.
Show-n-Tell Thursday
1. DBColumn and DBLookup for all browsers using AJAX
2. Using AJAX as a replacement for @DBLookups
I'm developing an application which needs uses quite a lot of formula (especially @DBLookup & @UserRoles of different selected person) at the client side. To port it to web, I need to use those too.
Instead of creating new agents for each @Formula, I created a formula setup Form where I can enter different formula. Each document will have a unique title (single word). I use tags (<keyn>) that will be replace by parameter pass in through url.

Using a view, I use a different form to open the document. This is set in the "Form Formula". The new form will compute the formula and return the result. In the view, set the title as the first column and sort it either ascending or descending. This way you can get the document using the title instead of document unique id
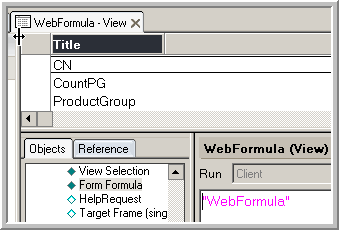
Below is a sample where different product group is selected, the products will be retrieved (DBLookup) and populated into the Product selection list. Check out previous post on dynamic selection list.

I use AJAX to call the formula document
<dbpath>/vWebFormula/<formula_document_title>?OpenDocument¶m=key1~value1
The param is optional. That will return the result in text format. You can then process it as needed. If you need more formula just create a new setup formula document and you are ready to use it. You can download the sample db here.
Show-n-Tell Thursday
Subscribe to:
Posts (Atom)